useReudcer hook takes the current state and returns a new state, it is used for state management. With useReducer, we can directly call the function inside the initialization and also can perform the functional tasks.
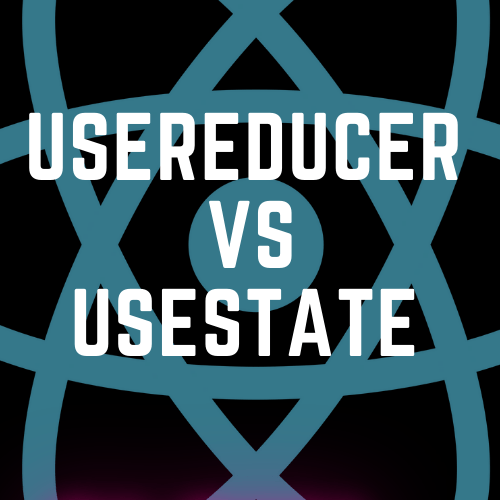
useReducer takes to arguments, previous state, and action, we perform actions to the previous state and get output as a new state.
useReducer is an alternative to the useState hook, infect useState build using useReducer.
If you ever worked with redux then you will understand useReducer easily it is the same functionality we used with redux reducers.
There is one javascript reducer function, useReducer is related to that, you can check this javascript reducer function here https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reduce
You must be thinking if useState and useReducer both are used for state management then why do we need two separate hooks for the same task, well there are some limitations with the useState hook
Limitation of useState Hook
if the logic of your code gets complicated then the setter function can be large in size and it will be difficult to handle it.
Also, you have to take care of returning new items in every setter function using the spreading operator. So every time you call useState with the object you have to use the spreading operator for managing previous values, which is very difficult.
Now let’s understand the differences.
Difference between useState and useReducer Hook (react useReducer vs useState)
useState hook is used for state transitions and dispatch useReducer is more likely used for state manipulation.
useState is mostly used when types of states are number, boolean, and string. useReducer is used when types of states are objects and arrays.
With useState we can handle only one or two-state transitions, but with useReducer, we can manage many state transitions. Also, state transitions are related in the case of useReducer.
When a more logical approach is required, it’s better you use useReducer.
useState hook use for the local component state, In the case of global state useReducer, is a better use.
useState and useReducer code examples to understand better
useState Hook Counter Example
In this example, we are creating a counter that increments and decrements on button click.
import React,{useState}from 'react';
const App = () => {
const [counter, setCounter] = useState(10);
return (
<>
<div>current counter is {counter}</div>
<button onClick={() => setCounter(counter+1)}>increase</button>
<button onClick={() => setCounter(counter-1)}>decrease</button>
</>
)
}
export default App;
In the above example, we can see if we put another value of a string or boolean or any type of variable into the state, we cannot prevent that from happening, and the effect on the state is scattered all over the place.
We cannot manage it in a proper way, it works better in the case of single values like numbers, and strings it’s better if we use useReducer in the case of objects and arrays.
Now let’s see the same example with useReducer.
useReducer Hook Counter Example
import React,{useReducer}from 'react';
const AppReducer = () => {
const [state, dispatchCounter] = useReducer((oldState,action)=>{
if(action.type=='inc'){
return {counter:oldState.counter+1}
}
if(action.type=='dec'){
return {counter:oldState.counter-1}
}
return oldState;
},
{counter:10});
return (
<>
<div>reducer current counter is {state.counter}</div>
<button onClick={() => dispatchCounter({type:'inc'})}>increase</button>
<button onClick={() => dispatchCounter({type:'dec'})}>decrease</button>
</>
)
}
export default AppReducer;
In the above code, the same counter process is happening of increasing and decreasing counter, but now you can pass any extra variable inside the previous state, and there will be no impact, also we have an old state and new state both for doing any calculation.
Finally, let me explain it once so you can understand it fully.
useReducer receives the previous state and must return to a new state, it also receives the action. Action is the value you call with your dispatch function, in the above code it’s dispatchCounter.
These was the major differences and explanation of useState vs useReducer.
Output for both examples will be the same
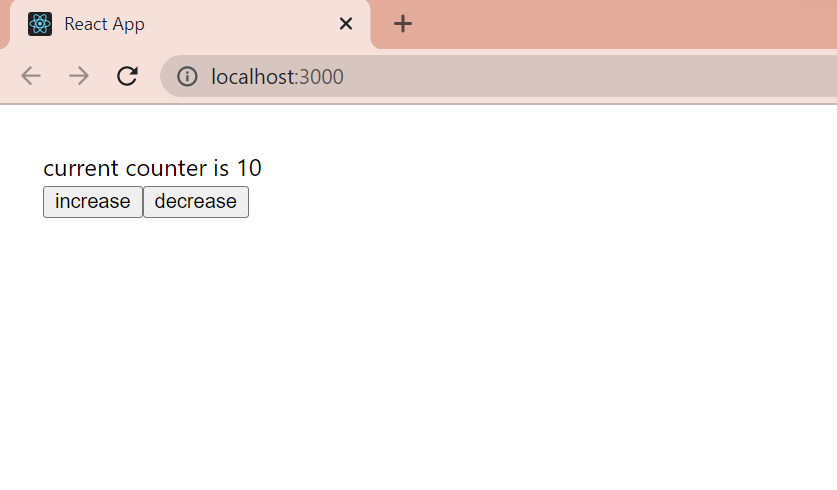
I hope you like this blog, share it with your friends who are learning React JS. Thank you 🙂