useEffect hook is used to manage side effects that are not related to component rendering. Mostly useEffect hook is used for managing console logs, animations, loading data, etc.
Let’s understand useEffect with this code.
import React,{useEffect, useState}from 'react';
const App = () => {
const [firstClick,setFirstClick] = useState(1);
const [secondClick,setSecondClick] = useState('2');
useEffect(() =>{
console.log(firstClick,secondClick);
});
return (
<>
<button onClick={() => setFirstClick(firstClick+1)}>Click 1</button>
<button onClick={() => setSecondClick('3')}>Click 2</button>
</>
)
}
export default App;
In the above code, we are creating two variables using useState for managing the state. the default value of these variables for one is an integer and for another one is a string.
firstClick variable is an integer and secondClick variable is a string.
By default, useEffect calls two times and it is called on all variable values changes. so if we click one button useEffect automatically calls and if we click another button useEffect gets called automatically.
What if we only want to call useEffect when there is a change in the value of the variable and also not for all the variables but for the specific one. like in this case if we only want to call useEffect when someone clicks on the Click 1 button.
Before we change our code to make useEffect calls for only one variable change, let’s see what happens in the above code.
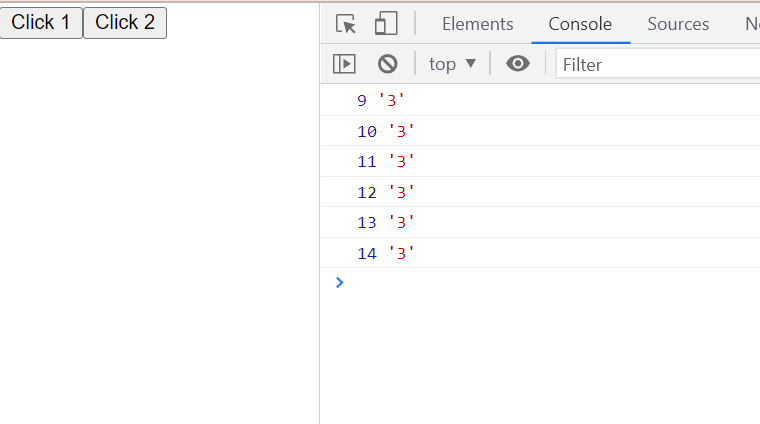
In the above code when the user clicks on the Click 1 button it gets incremented and on every click, we get the new value, so use effect calls every time when someone clicks on the Click 1 button, because we are getting a new value on every click and useEffect detects it.
But when we click on the Click 2 Button it only gets displayed once, because the secondClick variable is a string, and it’s not changing on every click and if there is no change, useEffect cannot be called.
Now let’s come to another question, how we can call useEffect only when there is a change in one variable, not on every button click.
Let’s understand with this example
import React,{useEffect, useState}from 'react';
const App = () => {
const [firstClick,setFirstClick] = useState(1);
const [secondClick,setSecondClick] = useState('2');
const [thirdClick,setThirdClick] = useState(50);
useEffect(() =>{
console.log(firstClick,secondClick,thirdClick);
});
return (
<>
<button onClick={() => setFirstClick(firstClick+1)}>Click 1</button>
<button onClick={() => setSecondClick('3')}>Click 2</button>
<button onClick={() => setThirdClick(thirdClick+1)}>Click 3</button>
</>
)
}
export default App;
In the above code, we have three variables now, firstClick, secondClick, and thirdClick.
The first and third variables are changing with every click so in this case, useEffect calls every time when someone clicks on the Click 1 and Click 3 buttons.
So useEffect not specifically detecting for one variable change here, so we have to find a way where only the Click 1 button can call useEffect, it should not call on clicks of the Click 3 button.
Well, there is an easy way, you just need to mention this variable inside the useEffect, format for this is.
useEffect(() =>{
console.log(firstClick,secondClick,thirdClick);
},[firstClick]);
So now, useEffect only calls when Click 1 button hits.
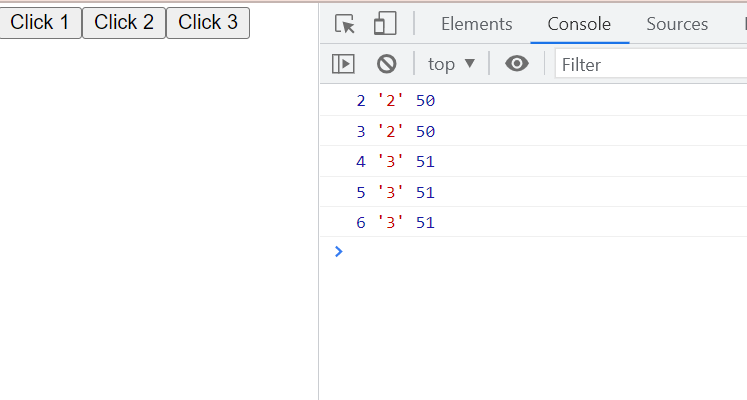
So it is now only calling when Click 1 button is pressed and for Click 2 and Click 3 buttons useEffect is not calling.
With this functionality, it is very useful for API calls, So whenever there is a change in filters we can call API request, or whenever there is a change in API request variable we can call API again.
this way we can make our project more responsive to API calls.
Not just API it is also useful for variable changes, if a variable changes and we have to perform an operation on it, useEffect can directly detect the change and do the necessary calculations.
useEffect is a very important hook I must say.
Fetching data from API using useEffect
For the API we are using jsonplaceholder.typicode.com free API.
import React,{useEffect, useState}from 'react';
const App = () => {
const [postCounter,setFirstClick] = useState(1);
const [commentCounter,setSecondClick] = useState(1);
useEffect(() =>{
console.log('https://jsonplaceholder.typicode.com/posts/'+postCounter);
fetch('https://jsonplaceholder.typicode.com/posts/'+postCounter)
.then(response => response.json())
.then(json => console.log(json))
},[postCounter]);
useEffect(() =>{
console.log('https://jsonplaceholder.typicode.com/comments/1'+commentCounter);
fetch('https://jsonplaceholder.typicode.com/comments/1'+commentCounter)
.then(response => response.json())
.then(json => console.log(json))
},[commentCounter]);
return (
<>
<button onClick={() => setFirstClick(postCounter+1)}>Get Posts</button>
<button onClick={() => setSecondClick(commentCounter+1)}>Get Comments</button>
</>
)
}
export default App;
In the above code, we have two buttons get Posts and get Comment on both clicks we are calling a useEffect function and we have two useEffect functions defined here and both are separately calling whenever there is a change in their respected variables.
On click of get Posts button postCounter incrementing and useEffect hook is calling, similarly on click of get Comments commentCounter variable is incrementing and useEffect hook is calling.
This way you can use useEffect for handling API calls.
You can use one useEffect and can handle API calls with if conditions or switch conditions.
Output for the above code is
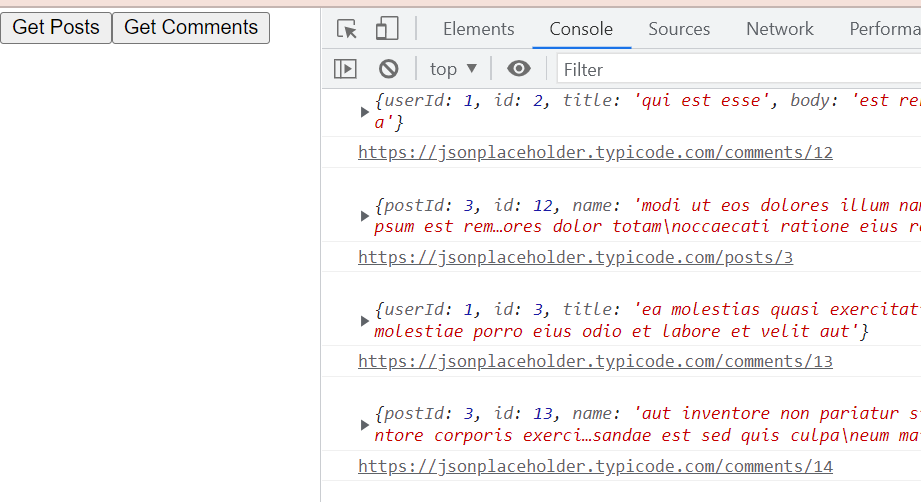
That’s it for this blog if you like please share, Thankyou 🙂