Hello everyone, welcome back! In today’s blog post, we’re going to explore arrays – an essential data structure in JavaScript that you’ve probably come across if you’ve done any coding. Whether you’re experienced in coding or just getting started, knowing about arrays is really important, especially if you’re preparing for interviews.
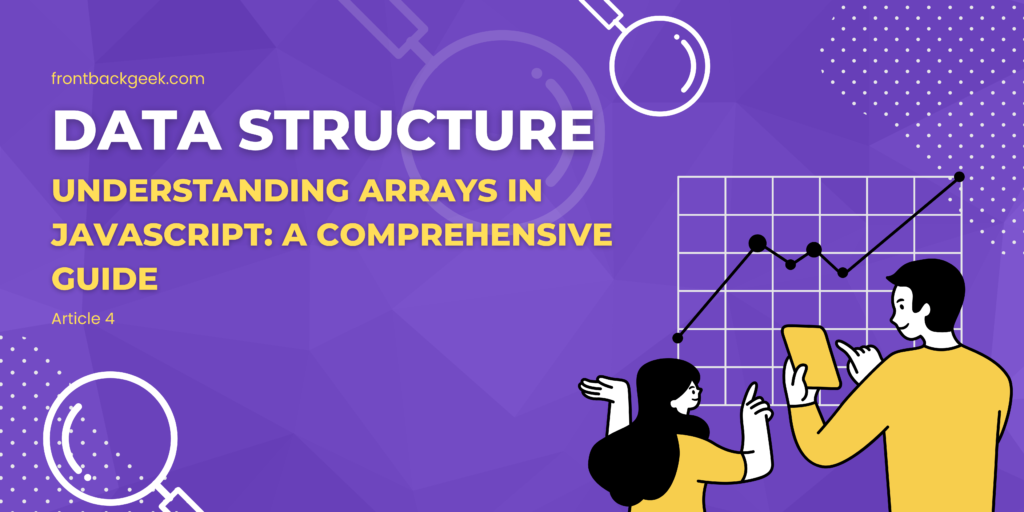
The Basics: Memory Allocation for Arrays
Let’s go back to the basics of how arrays are stored in memory. In a 32-bit system, each item in the array needs 4 bytes, and in a 64-bit system, it’s 8 bytes. For example, if we have an array called “box” with the numbers 1, 2, and 3, it would take up 12 memory slots (4 slots for each number).
Now, there are two types of arrays: static and dynamic. If you’ve used languages like C, C++, or Java, you probably know static arrays, where you have to say how big the array is when you create it. On the other hand, dynamic arrays, used in Python and JavaScript, handle the size automatically without needing you to say how big it is when you create it.
Knowing the difference between static and dynamic arrays is important because it affects how the computer uses and sets aside memory.
Common Operations on Arrays
Accessing Elements
Getting elements from an array is a basic thing we do. In JavaScript, doing this takes a constant amount of time, no matter how big the array is – we call this O(1) time and space complexity. The reason it’s so quick is because of how JavaScript organizes its memory. The formula for finding the value at a certain spot in the array is: starting memory slot + (index * size of each item).
// Accessing the second element in the 'box' array
const box = [1, 2, 3];
const secondElement = box[1]; // O(1) time complexity
Updating Elements
Changing or replacing a value in an array is also really quick – it’s a constant-time operation, O(1). The system figures out the memory address for the spot you want to change and updates the value right away.
// Updating the value at index 2 in the 'box' array
box[2] = 10; // O(1) time complexity
Traversing the Array
Going through every item in an array, which we call traversing, means using a loop. In JavaScript, this takes time that grows with the number of elements in the array – we call this linear time complexity, O(n), where n is how many items are in the array.
// Traversing the 'box' array
for (let i = 0; i < box.length; i++) {
console.log(box[i]); // O(n) time complexity
}
Searching for an Element
Searching for an element in an array is similar to traversal and also has a linear time complexity of O(n).
// Searching for the value '10' in the 'box' array
const searchValue = 10;
const foundIndex = box.indexOf(searchValue); // O(n) time complexity
Copying an Array
Copying an array means going through the whole array, and it takes time that increases with the number of elements – we call this O(n) time complexity. Also, it needs extra space, which makes it not very efficient, especially for big arrays.
// Copying the 'box' array
const copiedArray = [...box]; // O(n) time complexity
Inserting and Deleting Elements
Adding or removing things in an array can be quicker or slower depending on where you’re doing it.
If you’re adding something at the end, which is common, it’s usually quick – we call this O(1) in dynamic arrays because they can make room easily.
// Inserting a new element at the end of the 'box' array
box.push(20); // O(1) time complexity (amortized)
Removing something from the end of an array is also usually quick – we call this O(1) operation.
// Deleting the last element from the 'box' array
box.pop(); // O(1) time complexity
If you’re adding or removing something from the beginning or middle of an array, it takes longer. It involves moving items around, and we call this a O(n) operation because the time it takes grows with the number of elements in the array.
// Inserting a new element at the beginning of the 'box' array
box.unshift(5); // O(n) time complexity
// Deleting the first element from the 'box' array
box.shift(); // O(n) time complexity
Dynamic Arrays in JavaScript
Now, let’s talk about dynamic arrays in JavaScript. These arrays can change their size on their own, making it quicker to add things. The idea behind them is to set aside extra space, and it often follows the pattern of doubling the space each time.
// Example of dynamic array resizing
const dynamicArray = [100, 200, 300];
// System reserves space for 2^2 = 4 elements
dynamicArray.push(400); // No resizing needed (O(1))
// System reserves space for 2^3 = 8 elements
dynamicArray.push(500); // Resizing occurs, extra space for future elements (O(n))
Dynamic arrays bring a big benefit when it comes to adding things, especially when the array is getting bigger.
Conclusion
Knowing the details about how arrays work, where they store information in memory, and the challenges of common tasks is really important for any JavaScript developer. Whether you’re using static or dynamic arrays, understanding how they work helps you make smart choices based on what your code needs.
In the next part of this series, we’ll go even deeper into dynamic arrays and see how they affect how long things take in different situations. Keep an eye out for more insights into the world of data structures and JavaScript!
Thanks for sticking with us! See you in the next one. Happy Coding 🙂