Today, we’re going to talk about a very important part of making programs work well, called space complexity. We’ve looked at time complexity before, which is about how long a program takes to run. Now, we’re going to look at how much extra memory a program needs to work, besides the information it uses.
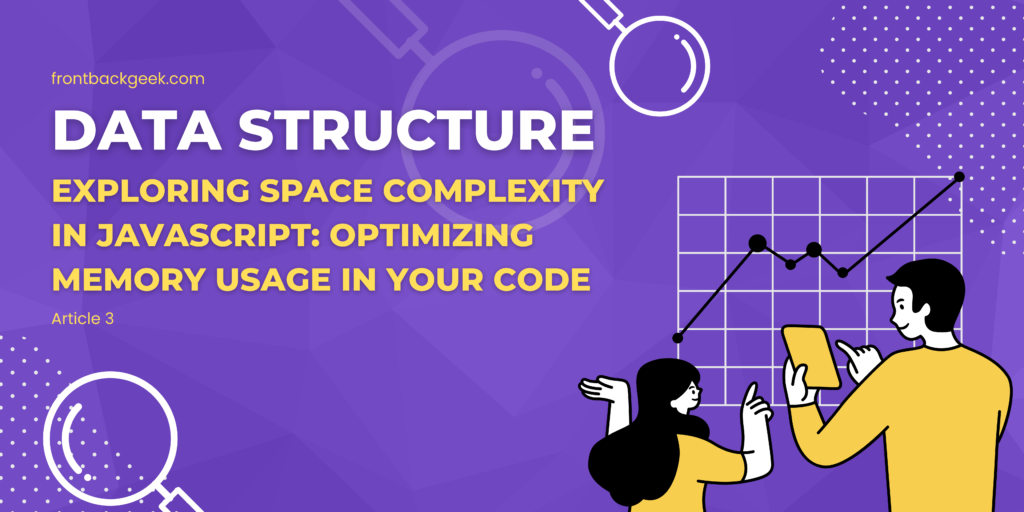
What is Space Complexity?
Let’s break down space complexity with some practical JavaScript examples.
Constant Space Complexity (O(1))
Consider a JavaScript function designed to calculate the cube of a number and print the result:
function printCube(num) {
const result = num * num * num;
console.log(result);
}
In this example, regardless of the input size, the function only needs a single additional space for the result
variable. This is a perfect illustration of constant space complexity, or O(1), where the extra memory required does not change with the input size.
Linear Space Complexity (O(n))
Now, let’s examine a scenario where space complexity is dependent on the input size:
function printCubes(list) {
const results = [];
for (let i = 0; i < list.length; i++) {
results.push(list[i] * list[i] * list[i]);
}
console.log(results);
}
Here, the results
array’s size grows linearly with the input list
length, demonstrating linear space complexity (O(n)). The more elements in the input list, the more space is required to store the calculation results.
Optimizing Space Complexity
Knowing how much extra space your programs need is key to making them run better and handle more information. This is really important when there’s not much memory available or when you’re working with a lot of data. By understanding how your code uses space, you can make smart choices to use less memory.
For example, changing your program so it doesn’t save lots of temporary results but calculates what it needs when it needs it can greatly decrease the amount of space it needs, from needing space that grows with the size of the data (O(n)) to needing a small, fixed amount of space (O(1)).
Conclusion
In summary, space complexity is a basic idea in programming that really affects how well and how much your applications can do. By understanding and using the ideas we talked about, and looking at the JavaScript examples as examples, you can get really good at spotting and making better the way your programs use memory. Remember, making a program work well isn’t just about making it fast, but also about using resources cleverly.
Thanks for sticking with us! Get into these ideas, try out the examples we gave, and see how you can make the memory use in your own projects better. Catch you in the next one!