Welcome to our discussion about linked lists, In this blog, we’ll talk about linked lists, an important thing in JavaScript for organizing data. If you’re new to data stuff or need a reminder, this blog is for you. We’ll talk about what linked lists are, why they matter compared to arrays, how they work, different types, and how they use memory.
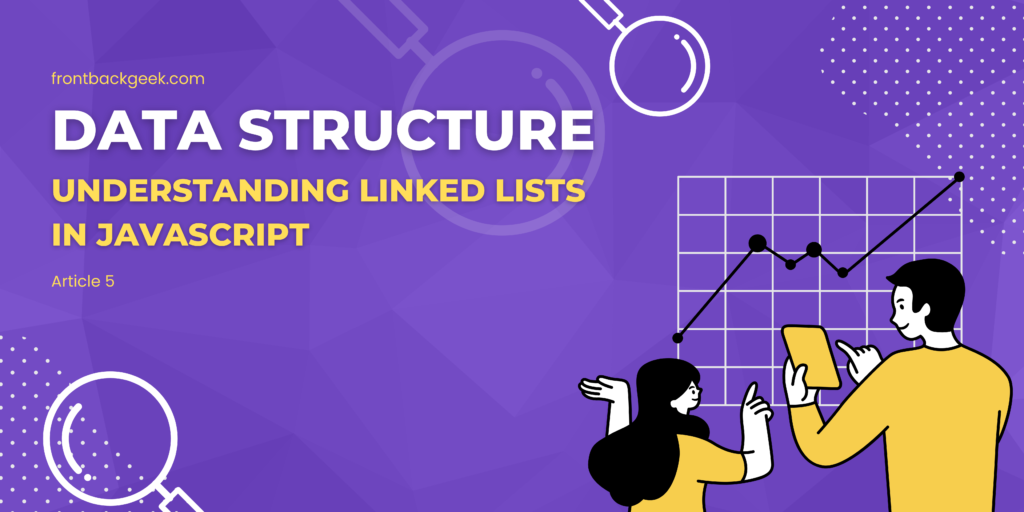
What are Linked Lists?
Linked lists are a basic data thing, like arrays. They’re important because they give a flexible way to store and organize data. Unlike arrays, which store elements in order in memory, linked lists store values differently. Let’s look at an example:
Array Representation
let array = [2, 5, 6];
In an array, elements are stored sequentially in memory like this
-------------------
| 2 | 5 | 6 | ... |
-------------------
Linked List Representation
// Node for the linked list
class Node {
constructor(data) {
this.data = data; // Value of the node
this.next = null; // Pointer to the next node
}
}
// Creating nodes for linked list
let node1 = new Node(2);
let node2 = new Node(5);
let node3 = new Node(6);
// Connecting the nodes
node1.next = node2;
node2.next = node3;
// Head of the linked list
let head = node1;
In a linked list, each element is a Node
with a value and a pointer to the next node
Node 1 Node 2 Node 3
+------+ +------+ +------+
| 2 | ----> | 5 | ----> | 6 |
+------+ +------+ +------+
Types of Linked Lists
Singly Linked List
In this type, each node points to the next node in the list.
You can move through the list in one direction, from the beginning (head) to the end (tail).
Doubly Linked List
Here, each node has pointers to both the next and previous nodes.
This allows you to move in both directions: from the start (head) to the end (tail) and back.
Now, let’s use some JavaScript code to show these ideas.
Circular Linked Lists
A Circular Linked List is like a singly linked list, but with one difference: instead of the last node pointing to null, it points back to the first node. This creates a circle where the last node connects back to the first.
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.