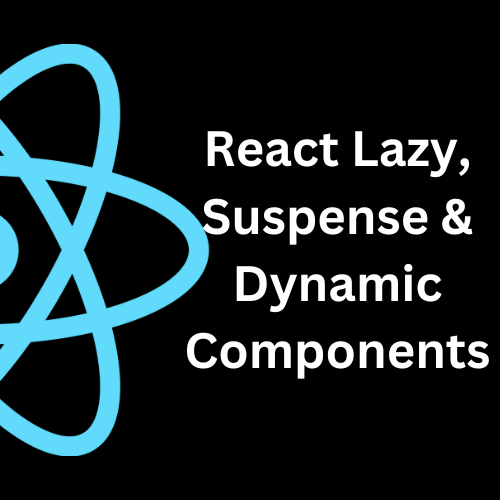
React Lazy and dynamic components, both are features of react that help in the lazy loading of components and also help in code splitting. They help to improve performance by only loading component when they are needed and this helps in reducing the bundle size of react project and also make the application much faster. Both sound similar but they have their different purposes which we are going to explain in a further article.
Let’s start with React Lazy.
React Lazy
React Lazy is a feature of React that enables the lazy loading of components. It dynamically loads components when needed.
If we have large components which are not needed immediately when the application starts then we can load them with the lazy load feature.
Example of React Lazy
Here we have two components, ComponentX and ComponentY, and we want to lazily load ComponentY.
The first step is to import the lazy function of React.
import React, { lazy, Suspense } from 'react';
Now in the next step, we can use the lazy function to wrap the dynamic import of ComponentY.
const ComponentY = lazy(() => import('./ComponentY'));
Now using a suspense component we can render ComponentY lazily and this suspense component allow a fallback UI while the lazy component is loading.
function App() {
return (
<div>
<h1>ComponentX</h1>
<Suspense fallback={<div>Loading...</div>}>
<ComponentY />
</Suspense>
<button onClick={() => console.log('Clicked!')}>Click Me</button>
</div>
);
}
From the above example, we can understand that the suspense component is showing div with a Loading… text till the ComponentY is fully rendered. This lazy rendering of the component and during this rendering time, showing an alternate fallback UI, shows the process of lazy loading.
What is Suspense in React
With the above example, we already got the idea of what is suspense right?
In short, Suspense in React is a component that allows us to add a fallback UI while waiting for some asynchronous content to load. It is mostly used for React Lazy loading components and asynchronous operations like data fetching from the API response.
So we can use it to show some loading images while we fetch data from the API.
Overall, Suspense in React makes it easy to manage the loading state and improve the user experience while dealing with the async response of content.
Dynamic Components
Dynamic components are basically not a feature of React, but you can use them as higher-order components (HOCs) or render props.
Dynamic components are basically using some technique in which you can render components based on some conditions.
Example usage of Dynamic Component (with render props):
function DynamicComponent({ condition, componentX, componentY }) {
return condition ? componentX() : componentY();
}
function App() {
return (
<DynamicComponent
condition={someCondition}
componentX={() => <ComponentX />}
componentY={() => <ComponentY />}
/>
);
}
So in the above example, we have ComponentX and ComponentY and both are rendering based on the condition.
We can use Dynamic components in react js when we need to display two different UI based on conditions, then dynamic component is a great choice and it is very easy to use. It’s not a feature of React, it’s a technique we can use to optimize the performance of React applications.
What is Higher order components (HOCs)
Higher Order Component is a function that takes a component as an argument and returns a new component. You can Resue component logic by using Higher-order components and this way you can make shared behavior and avoid duplication.
In our above code example, we were using the Higher-order components, we created a function Dynamic Component, which was a higher-order function. Then we call the Dynamic Component inside the App Component JSX and inside the condition props we executed some condition and on the basis of that condition or Dynamic component will behave, if the condition is true then this will return the ComponentX otherwise it will return ComponentY
Thats it for this article if you like it, please share it with your friends and Keep Smiling. Happy Coding 🙂