We want to build a high-performance web application and we want to optimize performance in React.js application, but its optimization and performance are the most crucial parts. If you apply effective optimization techniques then you can ensure smooth interactions with the application, decrease the loading time and enhance the user experience with the application.
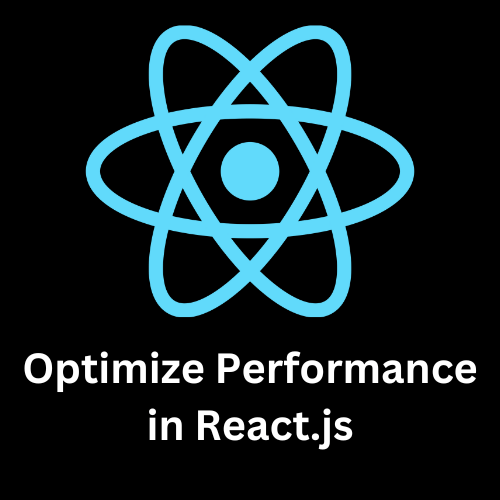
Here are some valuable tips that can help you to optimize the performance of your React.js application and make it fast in loading. Before tips and tricks, we need to clear some of the questions that come to our minds.
Why Optimize Performance in React.js Application Matters?
Performance optimization is very important in React.js as it impacts the user experience with the application.
Here are the key reasons why performance optimization is significant in React.js:
Enhanced User Experience and Increase Retention:
When you open a React.js application and it opens very fast, it creates a positive impact on the user experience. User will spend more time on it and this increase engagement with the application.
It helps to increase user satisfaction and retention rate.
Improved Conversion Rates:
There is no doubt about it If your application is better in performance and if it is responsive and loads fast, it is surely going to increase your conversion rates.
Performance-optimized applications can reduce bounce rates and abandoned transactions. If the application is smooth and fast it will enhance the customer experience of using it and if the customer is happy with your product quality and the hustle-free process of buying it, he will surely come back, which leads to high conversions.
SEO and Search Engines Rankings:
For the search engine like Google, one of the most important ranking factors is the page load speed of a website. Faster websites increase their ranks on search engines which leads to high organic traffic to the website.
Mobile Application like Experience:
React.js applications are mostly single-page applications, which behave like as smoothly as a mobile application running on your mobile. single page applications are less in bundle size when they are highly optimized. Mobile networks can have limited bandwidth and therefore less size of the application reduces unnecessary requests and improves the mobile experience.
Now let’s discuss the ways we can improve our React.js Application Performance.
Tips to Minimizing Render Cycles
If there are frequent changes inside multiple components then rendering can be an expensive operation. The only way to optimize rendering performance is by minimizing the unnecessary render cycles.
Techniques that include shouldComponentUpdate, PureComponent, and React.memo().
Let’s discuss each of these techniques:
shouldComponentUpdate Lifecycle Method
So basically, In React every time a component receives a new props or a state update, React by default re-renders the component and its children. So we need some method that can help us to decide, when we want to re-render the component and when we don’t want to re-render the component.
shouldComponentUpdate
The lifecycle method helps us to implement some logic and we can decide whether we want to update the component or not.
This method allows us to write the logic to check the current props and state and compare it with the next props and state and on that basis we can re-render our component. By default, shouldComponentUpdate
the method returns true.
Let’s see some code examples:
class MyComponent extends React.Component {
shouldComponentUpdate(nextProps, nextState) {
if (this.props.someProp === nextProps.someProp && this.state.someState === nextState.someState) {
return false; // Skip re-rendering
}
return true; // Re-render the component
}
render() {
return (
<div>
{/* Your code goes here */}
</div>
);
}
}
React.memo()
React’s memo()
is a higher-order component (HOC) that can be used with functional components. It memoizes the component based on its props and re-renders it only if the props have changed. It uses as a wrapper for a component.
When you wrap the component with React.memo then it will automatically compare the props and the next props and if there is no change in it, there will be no re-rendering will happen for the component.
Code example for React.memo():
import React from 'react';
const MyComponent = React.memo(({ someProp }) => {
// Render component UI
return (
<div>
{/* Your code goes here. */}
</div>
);
});
export default MyComponent;
In the above example, we can see that the component is wrapped in React.memo and will automatically check for the new state or props changes and update the component accordingly.
PureComponent:
PureComponents extend the functionality of regular components. PureComponent is a base class provided by React.
PureComponent automatically implements a shouldComponentUpdate
method to perform the shallow comparison of props and state with next props and state.
Always take care of that, PureComponent’s shallow comparison can be insufficient for deeply nested objects or arrays. for this, we need some memoization techniques using the useMemo()
hook.
Code example for creating a PureComponent:
class MyComponent extends React.PureComponent {
render() {
return (
<div>
{/* Your code goes here. */}
</div>
);
}
}
I hope you like this article, check out some of our important articles on React.js below
To understand state management better checkout this article.
What is Redux? What is action, reducer and store in Redux
1 Comment